// ES5의 함수 표현식
var es = function(){
console.log('hello');
}
es(); // 'hello'
// ES6의 화살표 함수 표현식
let es = () => {
console.log('hello');
}
es(); // 'hello'
함수내의 처리문이 하나면 중괄호를 생략할 수 있습니다.
let es = () => console.log('hello');
es(); // 'hello'
매개변수를 전달받아 리턴하는 과정도 간단해집니다.
// ES5의 매개변수 전달, 리턴
var add = function(a,b){
return a + b;
}
console.log( add(1,4) ); // 5
// ES6의 매개변수 전달, 리턴
let add = (a,b) => {
return a + b;
}
console.log( add(1,4) ); // 5
마찬가지로 처리문이 하나면 중괄호를 생략합니다.
let add = (a,b) => a + b;
console.log( add(1,4) ); // 5
화살표 함수의 this
let obj = {
num: 5,
add1: function(a){
console.log(this.num + a, this);
},
add2: (a) => {
console.log(this.num + a, this);
}
}
obj.add1(5);
obj.add2(5);
아래와 같은 결과가 나옵니다.
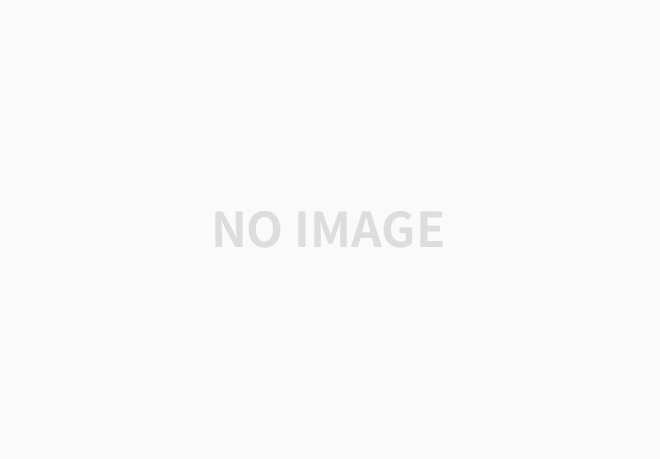
add1은 객체 내의 변수를 참조하지만 add2는 그 바깥쪽인 window 전역객체를 가르키므로
원하는 값을 리턴하지 못합니다.
this는 부모 객체를 바라봐야하는데 클로저가 생성되면 this가 바라보는 대상이 전역으로 변경되는 것과 비슷합니다.
let obj = {
num: 5,
add1: function(a){
console.log(this.num + a, this);
function inner(a){
console.log(this.num + a, this); // NaN, window
}
inner(5);
}
}
obj.add1(5); // 10, Object
화살표 함수를 사용하면 클로저에서 잘못된 this를 함수 범위내로 바라볼 수 있게 바꿀 수 있습니다.
let obj = {
num: 5,
add1: function(a){
console.log(this.num + a, this);
let inner = (a) => {
console.log(this.num + a, this);
}
inner(5);
}
}
obj.add1(5);
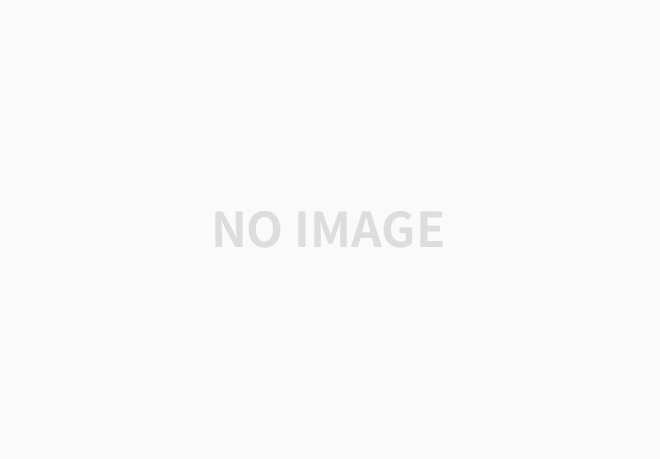
반응형
'javascript' 카테고리의 다른 글
filter() 함수 (0) | 2019.11.01 |
---|---|
map() 함수 (0) | 2019.10.28 |
로컬스토리지 예제 (0) | 2019.10.21 |
퀴즈 만들기 (0) | 2019.10.18 |
ES6의 클래스 (0) | 2019.10.17 |